In addition to supporting apps built for use while driving, Android Automotive OS supports browsers, games, and video apps for use while parked. You can ship the same app to cars as you do to other large screen devices with just a few minor changes.
Test your existing app on an Android Automotive OS emulator
To begin building your app for Android Automotive OS, first test your existing app on an Android Automotive OS emulator. To set up an emulator, follow the steps in Test using the Android Automotive OS emulator. You can then run the app by following the instructions in Run your app on the emulator.
When running your app, watch for compatibility issues, such as the following:
- Infotainment screens have fixed orientations. To meet the car app quality guidelines, apps must support both portrait and landscape orientations.
- APIs available on other devices might not be available on Android Automotive OS. For example, some Google Play Services APIs are not available on Android Automotive OS. See the Disable features section for details on how to handle these issues.
Configure your app's manifest files
To target Android Automotive OS, your app must have certain manifest entries. With them, apps targeting Android Automotive OS are submitted to the Play Store using a separate Automotive OS release type. They are put through a manual review process to help ensure that they're safe for use in a car. See Distribute Android apps for cars for more details.
Required Android Automotive OS features
To be listed in the Play Store in a car, apps built for Android Automotive OS
must include the following <uses-feature>
element in the AndroidManifest.xml
file:
<manifest ...>
...
<uses-feature
android:name="android.hardware.type.automotive"
android:required="true" />
...
</manifest>
Apps submitted to non-automotive tracks can't declare the <uses-feature>
element shown in the previous code sample, because they can't depend on
car-specific hardware. So, to ship the same app for both automotive and
non-automotive devices, you need to generate at least two flavors of your app:
one for automotive devices and another for mobile devices. For more information
about how to create these separate flavors, refer to the following documentation:
The two flavors of the app can share the same package name, but must have different version codes since they are uploaded to the Play Store tracks separately.
Alternatively, instead of using separate flavors, you can use separate package names for your mobile and automotive APKs or App Bundles. To understand the trade-offs of each approach, refer to Package names in the media app developer guide.
In addition to the element shown in the previous code sample, apps built for
Android Automotive OS must include the following <uses-feature>
elements in
the root <manifest>
element:
<uses-feature
android:name="android.hardware.wifi"
android:required="false"/>
<uses-feature
android:name="android.hardware.screen.portrait"
android:required="false"/>
<uses-feature
android:name="android.hardware.screen.landscape"
android:required="false"/>
Explicitly setting these features to not required helps ensure that your app doesn't conflict with available hardware features in Android Automotive OS devices.
Ensure there are no distraction-optimized activities
To ensure your app is only available for use while parked, don't
include the following <meta-data>
element in any
<activity>
element within your
manifest:
<!-- NOT ALLOWED -->
<meta-data
android:name="distractionOptimized"
android:value="true"/>
Without this metadata, your app's activities are blocked automatically
by the OS when the car enters driving mode, to reduce distractions for the
driver. This happens as an
onPause
lifecycle callback, during which you must pause both video and audio playback
from your app.
Category-specific manifest entries
In addition to the preceding requirements, which apply to all parked apps, the video and games categories have additional requirements:
- For video apps, see Mark your app as a video app.
- For games, see Mark your app as a game.
Optimize your app for Android Automotive OS
To give your users the best experience possible, keep the following things in mind while building your app for Android Automotive OS.
Optimize for large screens
The screens present in Android Automotive OS vehicles are more similar in size, resolution, and aspect ratio to tablets and foldables than to phones. As such, optimizing your app for large screens benefits your users in cars as well.
In particular, see the Support different screen sizes and Migrate your UI to responsive layouts guides for details on making the most of large display sizes, as well as the media and games galleries for design inspiration and guidance.
Other large screen optimizations such as input compatibility aren't as directly beneficial for Android Automotive OS, but they can still improve the user experience. For example, keyboard navigation makes use of the same APIs as rotary navigation, so any optimizations made there can benefit both form factors.
Work with window insets and display cutouts
As with other form factors, Android Automotive OS includes system UI elements, such as status and navigation bars, and support for non-rectangular displays.
By default, apps draw in an area that doesn't overlap with system bars or display cutouts. However, you might want your app to hide the system bars, draw content behind them, or show content in a display cutout as described in Lay out your app within window insets. If your app does any of these, refer to the following subsections for details on how to let your app work well across the ecosystem of Android Automotive OS devices.
System bars, immersive mode, and edge-to-edge rendering
System bars in cars may be sized and positioned differently than on other form factors. For example, navigation bars may be positioned on the left, right, or bottom of the screen. Even in the case that there is a status bar on top and a navigation bar on the bottom (as is the case with most phones and tablets), the size of these elements will likely be much greater in cars.
Additionally, Android Automotive OS allows OEMs to control whether or not
apps can show or hide the system bars to enter and exit immersive
mode. For example, by preventing apps from
hiding the system bars, OEMs can ensure that vehicle controls, such as climate
controls, are always accessible on screen. If an OEM has prevented apps from
controlling system bars, nothing happens when an app calls the
WindowInsetsController
(or WindowInsetsControllerCompat
)
APIs to show or hide system bars. Refer to the documentation of show
and
hide
to learn more about how to detect if your app was able to modify the
insets.
Likewise, OEMs can also control whether or not apps can set the color and translucency of system bars to ensure that the bars and the elements contained within them are clearly visible at all times. If your app draws edge-to-edge, check that only non-critical content is drawn behind system bars. This content may not be visible if the device OEM prevents setting the color or translucency of the bars.
<!-- Depending on OEM configuration, these style declarations
(and the corresponding runtime calls) may be ignored -->
<style name="...">
<item name="android:statusBarColor">...</item>
<item name="android:navigationBarColor">...</item>
<item name="android:windowTranslucentStatus">...</item>
<item name="android:windowTranslucentNavigation">...</status>
</style>
If your app goes edge-to-edge, don't make assumptions about the size, number, type, or location of system bars. Instead, use the window insets APIs to lay out your app's content relative to the system bars. See Display content edge-to-edge in your app for more details on how to use these APIs. Hard coded padding values that, while never recommended, may keep content in the safe area on other devices likely won't in cars.
Adapt to irregularly shaped displays
In addition to rectangular displays, some vehicles may have irregularly shaped screens, such as shown in Figure 1:
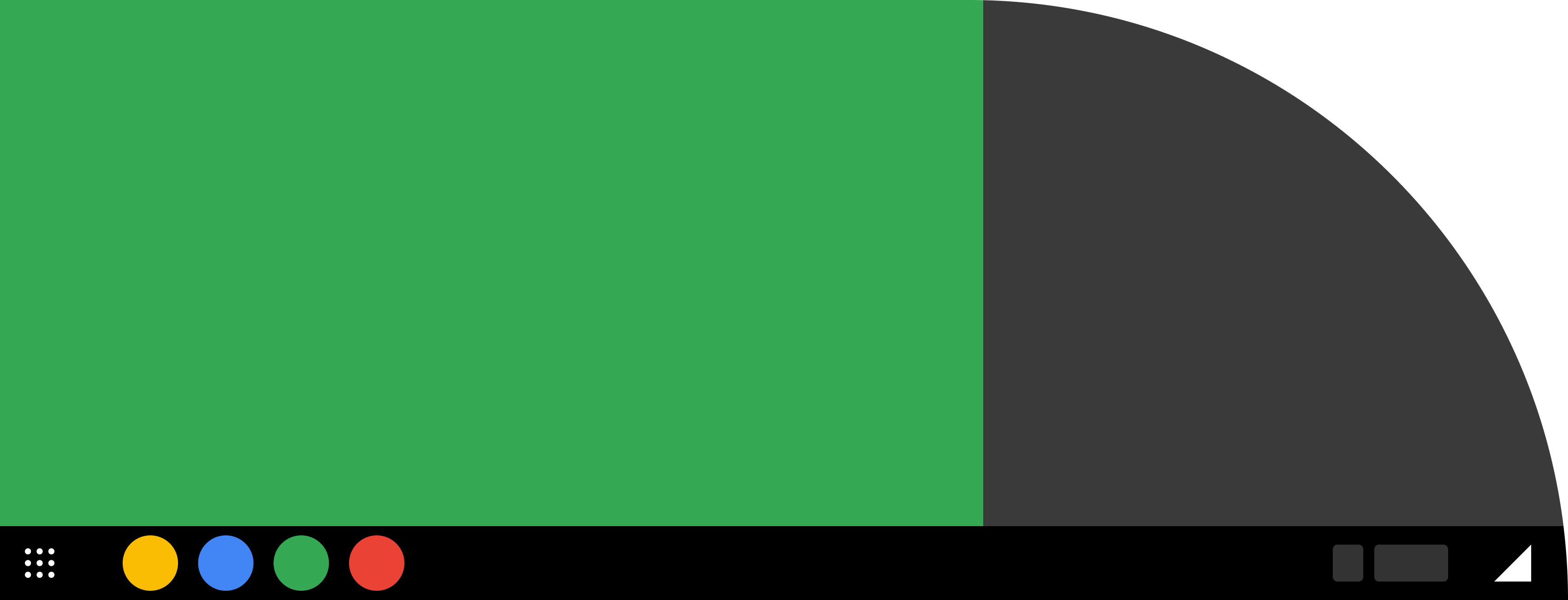
If your app doesn't render edge-to-edge, you don't need to do anything for it to render within the safe area.
If your app renders edge-to-edge, you can choose how you'd like it to
behave with respect to display cutouts. You can accomplish this using resources
by setting the
android:windowLayoutInDisplayCutoutMode
attribute for your app's theme or at runtime
by modifying the window's
layoutInDisplayCutoutMode
attribute.
Because the types of display cutouts present on Android Automotive OS devices
are different than those on mobile devices, don't use the
LAYOUT_IN_DISPLAY_CUTOUT_MODE_DEFAULT
or LAYOUT_IN_DISPLAY_CUTOUT_MODE_SHORT_EDGES
,
which have behavior optimized for the cutouts found on mobile devices. Instead,
use LAYOUT_IN_DISPLAY_CUTOUT_MODE_NEVER
or LAYOUT_IN_DISPLAY_CUTOUT_MODE_ALWAYS
to either always avoid or always enter the cutout. When choosing the latter,
see Support display cutouts for more
details on the APIs related to display cutouts.
If your app renders into the display cutout area and you'd like to have different behavior between Android Automotive OS and mobile, see Disable features for guidance if your app sets this behavior at runtime and Use alternate resources if your app sets this behavior using resource files.
Disable features
If you are making an existing mobile app available on Android Automotive OS, certain features and functionality might not be relevant or available. For example, cars generally don't provide access to cameras. Additionally, only a subset of Google Play services are available on Android Automotive OS; see Google Play services for cars for more details.
You can use the PackageManager.hasSystemFeature
API to detect whether the app is running on Android Automotive OS by checking
for the
FEATURE_AUTOMOTIVE
feature, as shown in the following example:
Kotlin
val packageManager: PackageManager = ... // Get a PackageManager from a Context val isCar = packageManager.hasSystemFeature(PackageManager.FEATURE_AUTOMOTIVE) if (isCar) { // Enable or disable a given feature }
Java
PackageManager packageManager = ... // Get a PackageManager from a Context boolean isCar = packageManager.hasSystemFeature(PackageManager.FEATURE_AUTOMOTIVE) if (isCar) { // Enable or disable a given feature }
Alternatively, if your app also has an Android Auto component, you can use the CarConnection API from the Android for Cars App Library to detect whether the app is running on Android Automotive OS or Android Auto—or if it is not connected to a car at all.
For Picture-in-Picture (PiP), follow the established best practices to check whether the feature is available and react appropriately.
Handle offline scenarios
While cars are becoming increasingly internet connected, apps are recommended to handle running without an internet connection, such as in the following cases:
- Users might opt out of mobile data offered as part of a subscription package from the auto maker.
- Access to mobile data might be limited in certain areas.
- Cars with Wi-Fi radios might be out of Wi-Fi range, or an OEM might turn off Wi-Fi in favor of a mobile network.
Be prepared to handle these scenarios in your app by gracefully degrading functionality that depends on internet access, such as by offering offline content. For more information, see the best practices for optimizing networking.
Use alternate resources
To help adapt your app for cars, you can use the car
resource qualifier to provide
alternate resources
when running on an Android Automotive OS vehicle. For example, if you use
Dimension resources to store
padding values, you could use a larger value for the car
resource set to make
touch targets larger.
Distribute your app
After you've tested your app against the car app quality guidelines for its category and made an Android Automotive OS build of it with any necessary changes for its category, you can then publish it to Automotive OS form factor tracks on the Play Store. See Distribute Android apps for cars for more details on the publishing process.
Give feedback on parked apps
If you run into an issue or have a feature request while developing your parked app for Android Automotive OS, you can report it using the Google Issue Tracker. Be sure to fill out all the requested information in the issue template. Before filing a new issue, check whether it is already reported in the issues list. You can subscribe and vote for issues by clicking the star for an issue in the tracker. For more information, see Subscribing to an Issue.