A display cutout is an area on some devices that extends into the display surface. It allows for an edge-to-edge experience while providing space for important sensors on the front of the device.
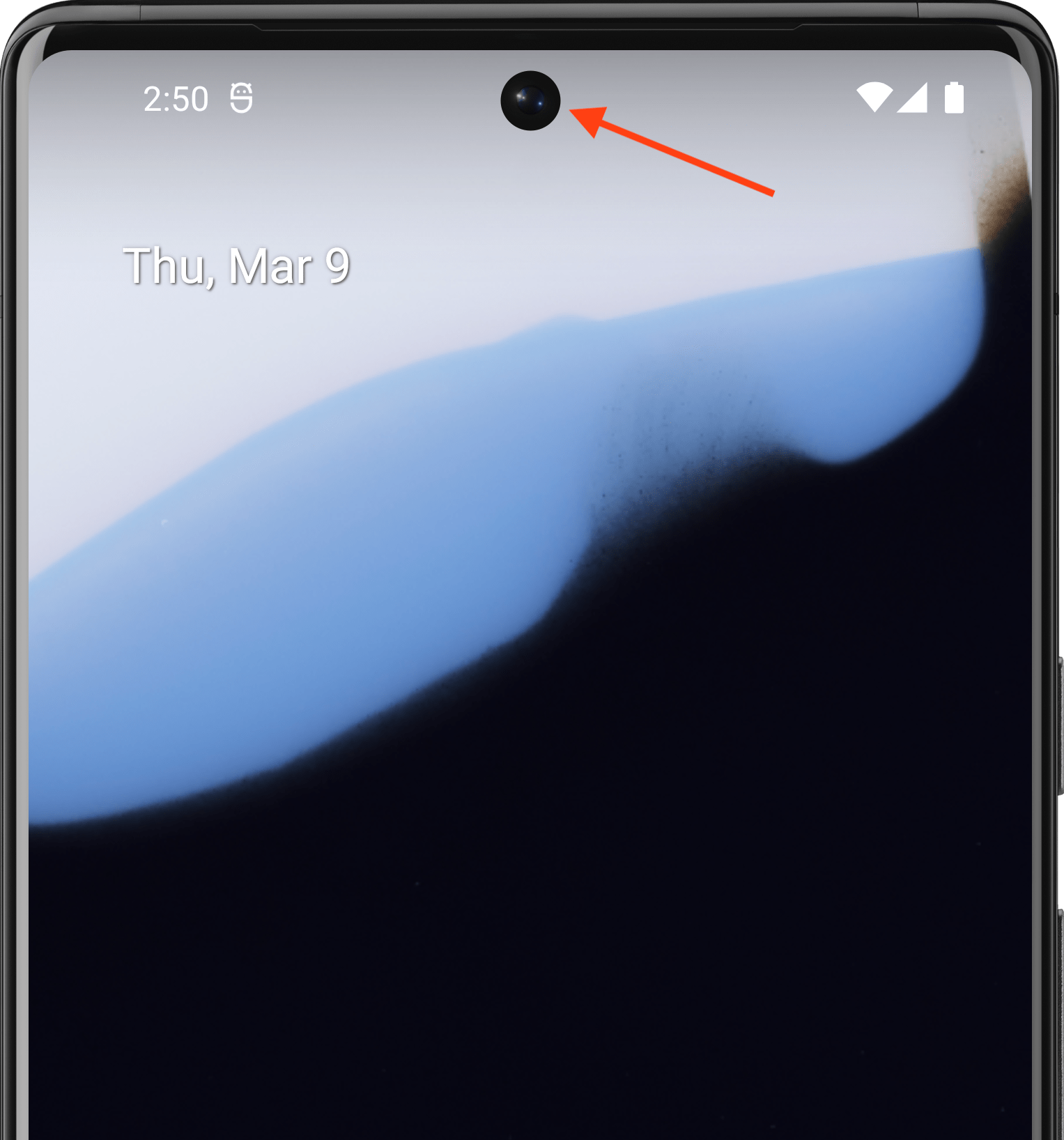
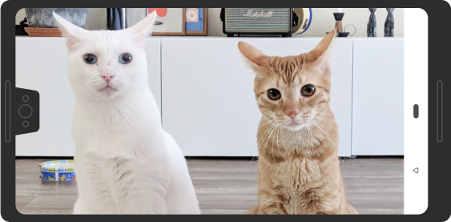
Android supports display cutouts on devices running Android 9 (API level 28) and higher. However, device manufacturers can also support display cutouts on devices running Android 8.1 or lower.
This page describes how to implement support for devices with cutouts in Compose, including how to work with the cutout area— that is, the edge-to-edge rectangle on the display surface that contains the cutout.
Default case
By default, display cutouts are included in the window insets information. Because of this, your app won't draw in the display cutout areas when you follow the guide on making your app edge-to-edge.
For example, when you use
Modifier.windowInsetsPadding(WindowInsets.safeContent)
or Modifier.windowInsetsPadding(WindowInsets.safeDrawing)
, your app
automatically won't draw in the areas where a cutout is placed.
WindowInsets.safeContent
and WindowInsets.safeDrawing
both contain display cutout information and will not draw where a device cutout
is.
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) WindowCompat.setDecorFitsSystemWindows(window, false) setContent { Box(Modifier.windowInsetsPadding(WindowInsets.safeContent)) { // Any composable inside here will avoid drawing behind cutouts } } }
To customize this behavior further, you need to handle the cutout information yourself.
Handle cutout information manually
You can handle cutouts in any of the following ways:
Setting in theme manifest with
android:windowLayoutInDisplayCutoutMode
Programmatically setting the option on a
Window
withwindow.attributes.layoutInDisplayCutoutMode
Accessing the cutout
Path
object withLocalView.current.rootWindowInsets.displayCutout
For Compose, it is recommended to set the windowLayoutInDisplayCutoutMode
to
default
in your overall theme, and then leverage WindowInsets.displayCutout
to handle the insets in your composables:
Canvas(modifier = Modifier.fillMaxSize().windowInsetsPadding(WindowInsets.displayCutout)) { drawRect(Color.Red, style = Stroke(2.dp.toPx())) }
This approach allows you to respect the displayCutout
padding where required,
or ignore it where it is not required.
Alternatively, you can apply the same settings that the Views Cutout
documentation describes by setting
either the activity theme android:windowLayoutInDisplayCutoutMode
to another
option, or setting the window attribute using
window.attributes.layoutInDisplayCutoutMode =
LAYOUT_IN_DISPLAY_CUTOUT_MODE_DEFAULT
. However, the cutout mode is then applied
to a whole activity, and cannot be controlled per individual composable.
To respect the display cutout in certain composables but not others, use
WindowInset.displayCutout
. This API allows you to access the cutout
information when required.
Best practices
When working with display cutouts, consider the following:
- Be mindful of the placement of critical elements of the UI. Don't let the cutout area obscure any important text, controls, or other information.
- Don't place or extend any interactive elements that require fine-touch recognition into the cutout area. Touch sensitivity might be lower in the cutout area.
- When following the edge-to-edge guidance, cutout information is included in
the
safeDrawing
/safeContent
insets. - Where possible, use
Modifier.windowInsetsPadding(WindowInsets.safeDrawing)
to determine the appropriate padding to apply to your content. Avoid hardcoding the status bar height, as this can lead to overlapping or cut-off content.
Test how your content renders with cutouts
Be sure to test all of your app's screens and experiences. Test on devices with different types of cutouts, if possible. If you don't have a device with a cutout, you can simulate common cutout configurations on any device or emulator running Android 9 or higher by doing the following:
- Enable Developer options.
- In the Developer options screen, scroll down to the Drawing section and select Simulate a display with a cutout.
- Select the cutout type.
Figure 3. Use Developer options to test how your content renders.
Recommended for you
- Note: link text is displayed when JavaScript is off
- Window insets in Compose
- Graphics Modifiers
- Style paragraph