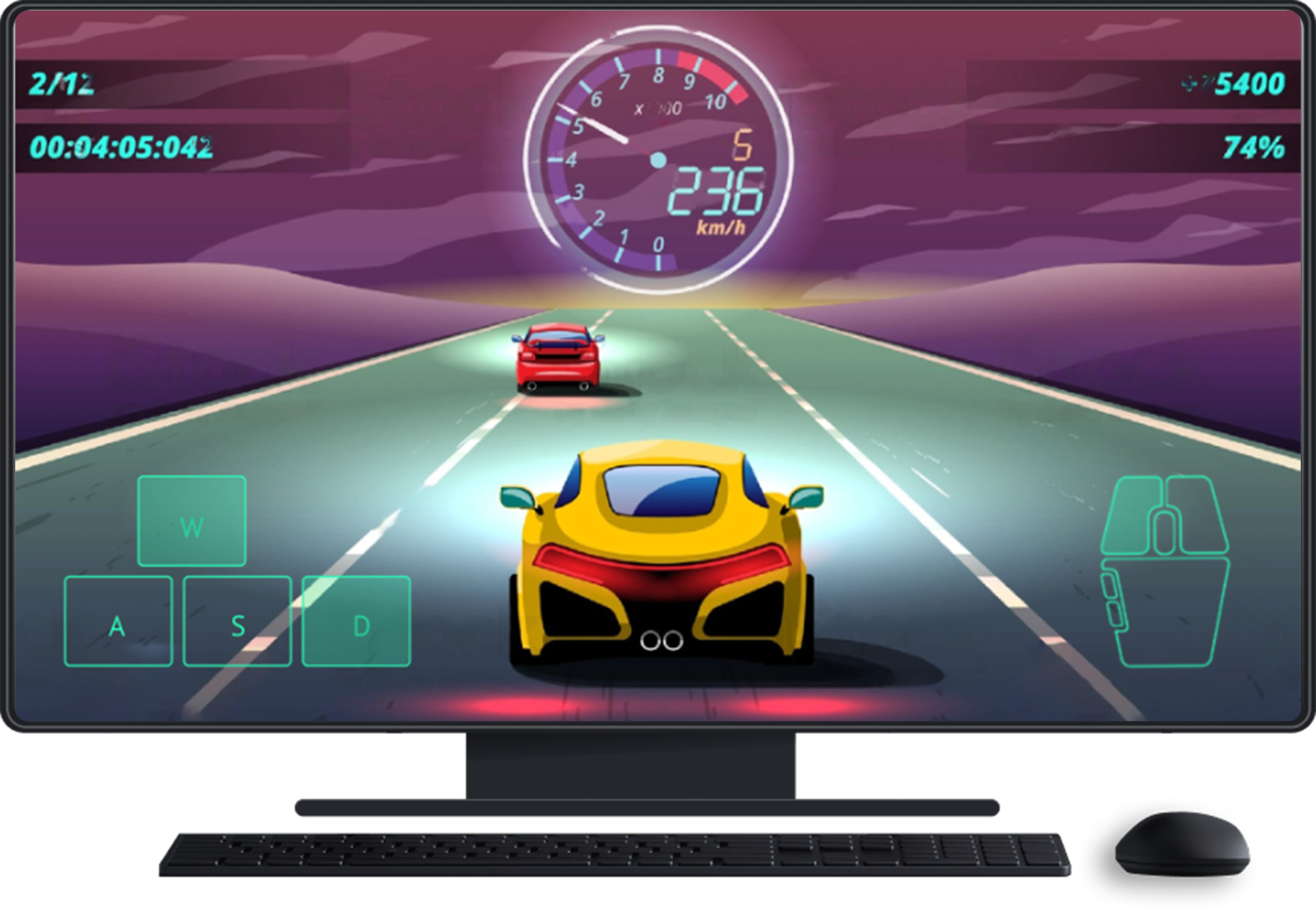
With new form factors come new forms of input. Touchscreen input works great on devices such as phones, foldables, tablets, and even some ChromeOS devices. However, make sure you cover the natural input cases for the form factors you're targeting.
Due to the prevalence of Google Play Games on PC and ChromeOS, quite a number of devices default to a desktop‑like form factor. This means keyboard and mouse input can be key to a comfortable and engaging user experience. If your game has a virtual joystick, D-pad, or on‑screen controls, map these actions to keyboard keys. This is especially important for games where speed of input or quick reflexes are critical for success, and also for games with complex touch gestures (like pinch and spread) or that require multiple touch inputs to comfortably play, as these inputs can't be simulated with just a cursor.
Support all form factors
Be sure that the input support work you do isn't locked to a specific form factor. Gamepads are used across all form factors. Players connect keyboards and mice to smartphones and tablets. Support these use cases to enable players to enjoy your game in the way they like playing best.