DrawableMarginSpan
public
class
DrawableMarginSpan
extends Object
implements
LeadingMarginSpan,
LineHeightSpan
java.lang.Object | |
↳ | android.text.style.DrawableMarginSpan |
A span which adds a drawable and a padding to the paragraph it's attached to.
If the height of the drawable is bigger than the height of the line it's attached to then the
line height is increased to fit the drawable. DrawableMarginSpan
allows setting a
padding between the drawable and the text. The default value is 0. The span must be set from the
beginning of the text, otherwise either the span won't be rendered or it will be rendered
incorrectly.
For example, a drawable and a padding of 20px can be added like this:
SpannableString string = new SpannableString("Text with a drawable.");
string.setSpan(new DrawableMarginSpan(drawable, 20), 0, string.length(),
Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
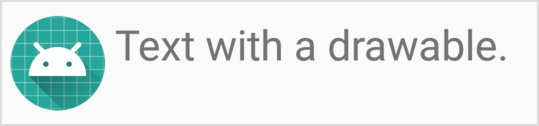
Summary
Public constructors | |
---|---|
DrawableMarginSpan(Drawable drawable)
Creates a |
|
DrawableMarginSpan(Drawable drawable, int pad)
Creates a |
Public methods | |
---|---|
void
|
chooseHeight(CharSequence text, int start, int end, int istartv, int v, Paint.FontMetricsInt fm)
Classes that implement this should define how the height is being calculated. |
void
|
drawLeadingMargin(Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. |
Drawable
|
getDrawable()
Returns the drawable used. |
int
|
getLeadingMargin(boolean first)
Returns the amount by which to adjust the leading margin. |
int
|
getPadding()
Returns a distance between the drawable and text in pixel. |
String
|
toString()
Returns a string representation of the object. |
Inherited methods | |
---|---|
Public constructors
DrawableMarginSpan
public DrawableMarginSpan (Drawable drawable)
Creates a DrawableMarginSpan
from a Drawable
. The pad width will be 0.
Parameters | |
---|---|
drawable |
Drawable : the drawable to be added
This value cannot be null . |
DrawableMarginSpan
public DrawableMarginSpan (Drawable drawable, int pad)
Creates a DrawableMarginSpan
from a Drawable
and a padding, in pixels.
Parameters | |
---|---|
drawable |
Drawable : the drawable to be added
This value cannot be null . |
pad |
int : the distance between the drawable and the text |
Public methods
chooseHeight
public void chooseHeight (CharSequence text, int start, int end, int istartv, int v, Paint.FontMetricsInt fm)
Classes that implement this should define how the height is being calculated.
Parameters | |
---|---|
text |
CharSequence : This value cannot be null . |
start |
int : the start of the line |
end |
int : the end of the line |
istartv |
int : the start of the span |
v |
int : the line height |
fm |
Paint.FontMetricsInt : This value cannot be null . |
drawLeadingMargin
public void drawLeadingMargin (Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. This is called before the margin has been
adjusted by the value returned by getLeadingMargin(boolean)
.
Parameters | |
---|---|
c |
Canvas : This value cannot be null . |
p |
Paint : This value cannot be null . |
x |
int : the current position of the margin |
dir |
int : the base direction of the paragraph; if negative, the margin
is to the right of the text, otherwise it is to the left. |
top |
int : the top of the line |
baseline |
int : the baseline of the line |
bottom |
int : the bottom of the line |
text |
CharSequence : This value cannot be null . |
start |
int : the start of the line |
end |
int : the end of the line |
first |
boolean : true if this is the first line of its paragraph |
layout |
Layout : This value cannot be null . |
getDrawable
public Drawable getDrawable ()
Returns the drawable used.
Returns | |
---|---|
Drawable |
a drawable
This value cannot be null . |
getLeadingMargin
public int getLeadingMargin (boolean first)
Returns the amount by which to adjust the leading margin. Positive values move away from the leading edge of the paragraph, negative values move towards it.
Parameters | |
---|---|
first |
boolean : true if the request is for the first line of a paragraph,
false for subsequent lines |
Returns | |
---|---|
int |
the offset for the margin. |
getPadding
public int getPadding ()
Returns a distance between the drawable and text in pixel.
This units of this value are pixels.
Returns | |
---|---|
int |
a distance pixel from the text This units of this value are pixels. {} |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |