ImageSpan
public
class
ImageSpan
extends DynamicDrawableSpan
java.lang.Object | |||||
↳ | android.text.style.CharacterStyle | ||||
↳ | android.text.style.MetricAffectingSpan | ||||
↳ | android.text.style.ReplacementSpan | ||||
↳ | android.text.style.DynamicDrawableSpan | ||||
↳ | android.text.style.ImageSpan |
Span that replaces the text it's attached to with a Drawable
that can be aligned with
the bottom or with the baseline of the surrounding text. The drawable can be constructed from
varied sources:
Bitmap
- seeImageSpan(android.content.Context, android.graphics.Bitmap)
andImageSpan(android.content.Context, android.graphics.Bitmap, int)
Drawable
- seeImageSpan(android.graphics.drawable.Drawable, int)
- resource id - see
ImageSpan(android.content.Context, int, int)
Uri
- seeImageSpan(android.content.Context, android.net.Uri, int)
DynamicDrawableSpan#ALIGN_BOTTOM
For example, an ImagedSpan
can be used like this:
SpannableString string = new SpannableString("Bottom: span.\nBaseline: span."); // using the default alignment: ALIGN_BOTTOM string.setSpan(new ImageSpan(this, R.mipmap.ic_launcher), 7, 8, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE); string.setSpan(new ImageSpan(this, R.mipmap.ic_launcher, DynamicDrawableSpan.ALIGN_BASELINE), 22, 23, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
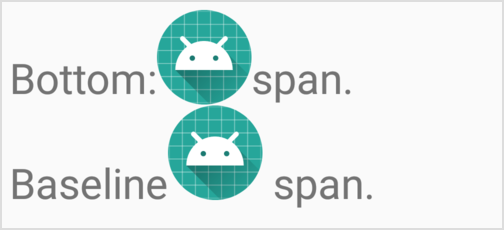
ImageSpan
s aligned bottom and baseline.Summary
Inherited constants |
---|
Inherited fields |
---|
Public constructors | |
---|---|
ImageSpan(Bitmap b)
This constructor is deprecated.
Use |
|
ImageSpan(Bitmap b, int verticalAlignment)
This constructor is deprecated.
Use |
|
ImageSpan(Context context, Bitmap bitmap)
Constructs an |
|
ImageSpan(Context context, Bitmap bitmap, int verticalAlignment)
Constructs an |
|
ImageSpan(Drawable drawable)
Constructs an |
|
ImageSpan(Drawable drawable, int verticalAlignment)
Constructs an |
|
ImageSpan(Drawable drawable, String source)
Constructs an |
|
ImageSpan(Drawable drawable, String source, int verticalAlignment)
Constructs an |
|
ImageSpan(Context context, Uri uri)
Constructs an |
|
ImageSpan(Context context, Uri uri, int verticalAlignment)
Constructs an |
|
ImageSpan(Context context, int resourceId)
Constructs an |
|
ImageSpan(Context context, int resourceId, int verticalAlignment)
Constructs an |
Public methods | |
---|---|
Drawable
|
getDrawable()
Your subclass must implement this method to provide the bitmap to be drawn. |
String
|
getSource()
Returns the source string that was saved during construction. |
String
|
toString()
Returns a string representation of the object. |
Inherited methods | |
---|---|
Public constructors
ImageSpan
public ImageSpan (Bitmap b)
This constructor is deprecated.
Use ImageSpan(android.content.Context, android.graphics.Bitmap)
instead.
Parameters | |
---|---|
b |
Bitmap : This value cannot be null . |
ImageSpan
public ImageSpan (Bitmap b, int verticalAlignment)
This constructor is deprecated.
Use ImageSpan(android.content.Context, android.graphics.Bitmap, int)
instead.
Parameters | |
---|---|
b |
Bitmap : This value cannot be null . |
verticalAlignment |
int |
ImageSpan
public ImageSpan (Context context, Bitmap bitmap)
Constructs an ImageSpan
from a Context
and a Bitmap
with the default
alignment DynamicDrawableSpan#ALIGN_BOTTOM
Parameters | |
---|---|
context |
Context : context used to create a drawable from based on the display
metrics of the resources
This value cannot be null . |
bitmap |
Bitmap : bitmap to be rendered
This value cannot be null . |
ImageSpan
public ImageSpan (Context context, Bitmap bitmap, int verticalAlignment)
Constructs an ImageSpan
from a Context
, a Bitmap
and a vertical
alignment.
Parameters | |
---|---|
context |
Context : context used to create a drawable from based on
the display metrics of the resources
This value cannot be null . |
bitmap |
Bitmap : bitmap to be rendered
This value cannot be null . |
verticalAlignment |
int : one of DynamicDrawableSpan#ALIGN_BOTTOM or
DynamicDrawableSpan#ALIGN_BASELINE |
ImageSpan
public ImageSpan (Drawable drawable)
Constructs an ImageSpan
from a drawable with the default
alignment DynamicDrawableSpan#ALIGN_BOTTOM
.
Parameters | |
---|---|
drawable |
Drawable : drawable to be rendered
This value cannot be null . |
ImageSpan
public ImageSpan (Drawable drawable, int verticalAlignment)
Constructs an ImageSpan
from a drawable and a vertical alignment.
Parameters | |
---|---|
drawable |
Drawable : drawable to be rendered
This value cannot be null . |
verticalAlignment |
int : one of DynamicDrawableSpan#ALIGN_BOTTOM or
DynamicDrawableSpan#ALIGN_BASELINE |
ImageSpan
public ImageSpan (Drawable drawable, String source)
Constructs an ImageSpan
from a drawable and a source with the default
alignment DynamicDrawableSpan#ALIGN_BOTTOM
Parameters | |
---|---|
drawable |
Drawable : drawable to be rendered
This value cannot be null . |
source |
String : drawable's Uri source
This value cannot be null . |
ImageSpan
public ImageSpan (Drawable drawable, String source, int verticalAlignment)
Constructs an ImageSpan
from a drawable, a source and a vertical alignment.
Parameters | |
---|---|
drawable |
Drawable : drawable to be rendered
This value cannot be null . |
source |
String : drawable's uri source
This value cannot be null . |
verticalAlignment |
int : one of DynamicDrawableSpan#ALIGN_BOTTOM or
DynamicDrawableSpan#ALIGN_BASELINE |
ImageSpan
public ImageSpan (Context context, Uri uri)
Constructs an ImageSpan
from a Context
and a Uri
with the default
alignment DynamicDrawableSpan#ALIGN_BOTTOM
. The Uri source can be retrieved via
getSource()
Parameters | |
---|---|
context |
Context : context used to create a drawable from based on the display
metrics of the resources
This value cannot be null . |
uri |
Uri : Uri used to construct the drawable that will be rendered
This value cannot be null . |
ImageSpan
public ImageSpan (Context context, Uri uri, int verticalAlignment)
Constructs an ImageSpan
from a Context
, a Uri
and a vertical
alignment. The Uri source can be retrieved via getSource()
Parameters | |
---|---|
context |
Context : context used to create a drawable from based on
the display
metrics of the resources
This value cannot be null . |
uri |
Uri : Uri used to construct the drawable that will be rendered.
This value cannot be null . |
verticalAlignment |
int : one of DynamicDrawableSpan#ALIGN_BOTTOM or
DynamicDrawableSpan#ALIGN_BASELINE |
ImageSpan
public ImageSpan (Context context, int resourceId)
Constructs an ImageSpan
from a Context
and a resource id with the default
alignment DynamicDrawableSpan#ALIGN_BOTTOM
Parameters | |
---|---|
context |
Context : context used to retrieve the drawable from resources
This value cannot be null . |
resourceId |
int : drawable resource id based on which the drawable is retrieved |
ImageSpan
public ImageSpan (Context context, int resourceId, int verticalAlignment)
Constructs an ImageSpan
from a Context
, a resource id and a vertical
alignment.
Parameters | |
---|---|
context |
Context : context used to retrieve the drawable from resources
This value cannot be null . |
resourceId |
int : drawable resource id based on which the drawable is retrieved. |
verticalAlignment |
int : one of DynamicDrawableSpan#ALIGN_BOTTOM or
DynamicDrawableSpan#ALIGN_BASELINE |
Public methods
getDrawable
public Drawable getDrawable ()
Your subclass must implement this method to provide the bitmap to be drawn. The dimensions of the bitmap must be the same from each call to the next.
Returns | |
---|---|
Drawable |
getSource
public String getSource ()
Returns the source string that was saved during construction.
Returns | |
---|---|
String |
the source string that was saved during construction
This value may be null . |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |