AlignmentSpan.Standard
public
static
class
AlignmentSpan.Standard
extends Object
implements
AlignmentSpan,
ParcelableSpan
java.lang.Object | |
↳ | android.text.style.AlignmentSpan.Standard |
Default implementation of the AlignmentSpan
.
For example, a text written in a left to right language, like English, which is by default aligned to the left, can be aligned opposite to the layout direction like this:
SpannableString string = new SpannableString("Text with opposite alignment");
string.setSpan(new AlignmentSpan.Standard(Layout.Alignment.ALIGN_OPPOSITE), 0,
string.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
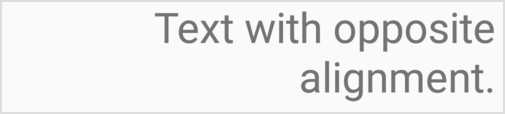
A text written in a right to left language, like Hebrew, which is by default aligned to the right, can be aligned opposite to the layout direction like this:
SpannableString string = new SpannableString("\u05d8\u05e7\u05e1\u05d8 \u05e2\u05dd \u05d9\u05d9\u05e9\u05d5\u05e8 \u05d4\u05e4\u05d5\u05da");
string.setSpan(new AlignmentSpan.Standard(Layout.Alignment.ALIGN_OPPOSITE), 0,
string.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
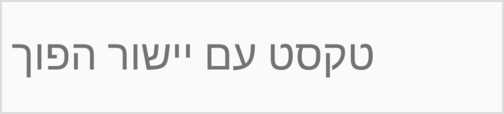
Summary
Inherited constants |
---|
Public constructors | |
---|---|
Standard(Parcel src)
Constructs a |
|
Standard(Layout.Alignment align)
Constructs a |
Public methods | |
---|---|
int
|
describeContents()
Describe the kinds of special objects contained in this Parcelable instance's marshaled representation. |
Layout.Alignment
|
getAlignment()
Returns the alignment of the text. |
int
|
getSpanTypeId()
Return a special type identifier for this span class. |
String
|
toString()
Returns a string representation of the object. |
void
|
writeToParcel(Parcel dest, int flags)
Flatten this object in to a Parcel. |
Inherited methods | |
---|---|
Public constructors
Standard
public Standard (Parcel src)
Constructs a Standard
from a parcel.
Parameters | |
---|---|
src |
Parcel : This value cannot be null . |
Standard
public Standard (Layout.Alignment align)
Constructs a Standard
from an alignment.
Parameters | |
---|---|
align |
Layout.Alignment : This value cannot be null . |
Public methods
describeContents
public int describeContents ()
Describe the kinds of special objects contained in this Parcelable
instance's marshaled representation. For example, if the object will
include a file descriptor in the output of writeToParcel(android.os.Parcel, int)
,
the return value of this method must include the
CONTENTS_FILE_DESCRIPTOR
bit.
Returns | |
---|---|
int |
a bitmask indicating the set of special object types marshaled
by this Parcelable object instance.
Value is either 0 or CONTENTS_FILE_DESCRIPTOR |
getAlignment
public Layout.Alignment getAlignment ()
Returns the alignment of the text.
Returns | |
---|---|
Layout.Alignment |
the text alignment |
getSpanTypeId
public int getSpanTypeId ()
Return a special type identifier for this span class.
Returns | |
---|---|
int |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |
writeToParcel
public void writeToParcel (Parcel dest, int flags)
Flatten this object in to a Parcel.
Parameters | |
---|---|
dest |
Parcel : This value cannot be null . |
flags |
int : Additional flags about how the object should be written.
May be 0 or Parcelable.PARCELABLE_WRITE_RETURN_VALUE .
Value is either 0 or a combination of Parcelable.PARCELABLE_WRITE_RETURN_VALUE , and android.os.Parcelable.PARCELABLE_ELIDE_DUPLICATES |